Gen AI PPT Maker
- Sairam Penjarla
- Dec 8, 2024
- 7 min read
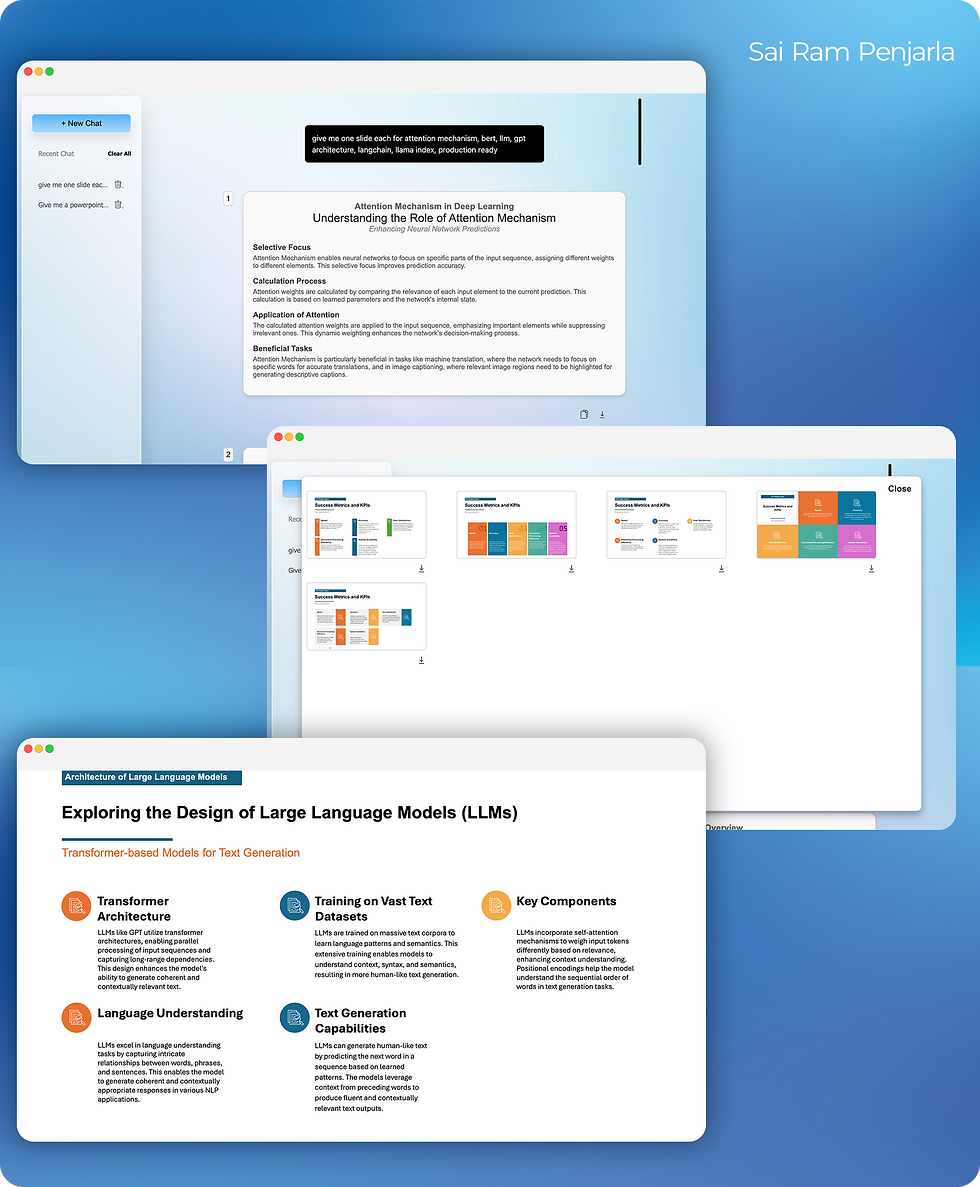
Watch this video to learn more about the project: [YouTube Video Placeholder]
Theory Section
Before diving into the implementation, let's understand the core concepts and technologies behind this project. The Gen AI PPT Maker project utilizes several technologies and tools to generate and manage PowerPoint presentations through AI. Here are some key elements to help explain the theory:
OpenAI GPT Model:
This project uses OpenAI's GPT models to generate textual content for each slide based on user input.
The GPT model can understand prompts given by the user and create detailed descriptions, titles, and content for the presentation slides.
Flask:
Flask is a Python web framework used for building the backend of this project.
It handles the routing, user sessions, and serves as the main interface for interacting with the AI engine.
HTML, CSS, JavaScript:
The frontend is designed using standard web technologies like HTML, CSS, and JavaScript.
The user interface allows users to input their requests, choose designs, and download generated presentations.
SQLite:
SQLite is used for storing session data, user inputs, and logs.
The project keeps track of all past sessions, making it easy to revisit previous presentations.
PowerPoint Templates:
The project allows users to select designs for their slides from a catalog.
Custom templates can be added by uploading PowerPoint files with predefined text elements mapped to certain names (e.g., “slide_title”, “content1”, etc.).
Environment Setup:
The project requires the user to set up an .env file with the OpenAI API token for the AI model to function.
Project Implementation
The Gen AI PPT Maker is an application designed to generate PowerPoint presentations using AI. Here’s an overview of how the project works:
User Interaction:
Users can input details like the number of slides, content requirements, and slide topics.
Based on this input, the AI generates text for each slide. Users can specify text for titles, headings, and body content.
Slide Generation:
After receiving input, the AI uses the OpenAI model to generate text content for each slide.
The generated content is displayed on the web interface, where users can review it.
Template Selection and Download:
Users can select a design template from a catalog.
After the AI generates the slides, users can download a single PowerPoint slide with the chosen design and AI-generated text.
Session Tracking:
Every user interaction is stored in the system, and past sessions are accessible through the sidebar.
Logs of interactions are saved in the app.log file for troubleshooting or reviewing past activity.
Custom Template Addition:
Users can add new templates by uploading PowerPoint files with predefined text elements.
Each text element in the template must be mapped to a name like “slide_title” or “content1”, allowing the AI to replace the text with its generated content.
Project Setup Instructions
To get started with the Gen AI PPT Maker project, follow these setup instructions:
Clone the Repository:
First, clone the repository from GitHub:
git clone <https://github.com/sairam-penjarla/genai-ppt-maker-flask>
Navigate to the Project Directory:
Change into the project directory:
cd genai-ppt-maker-flask
Create a Virtual Environment:
If you're unfamiliar with setting up a virtual environment, you can learn more in my blog post: Learn VirtualEnv Basics.
For a basic virtual environment setup, run the following:
python3 -m venv venv
Activate the Virtual Environment:
On Windows:
venv\\Scripts\\activate
On macOS/Linux:
source venv/bin/activate
Install Dependencies:
Install the required dependencies using the requirements.txt file:
pip install -r requirements.txt
Set Up Environment Variables:
Create a .env file in the project root directory and add your OpenAI API token:
OPENAI_API_TOKEN=your_api_token_here
Run the Project:
Start the Flask application:
python app.py
The application should now be running locally. Open your browser and navigate to http://127.0.0.1:5000/ to use the application.
Detailed Code Walkthrough
1. Imports and Setup
Explanation:
This block imports various necessary libraries and modules that the application will use. The project utilizes Flask for web application development, along with utilities for session management, logging, and PowerPoint generation. The json, pptx, and traceback libraries help in handling JSON data, manipulating PowerPoint slides, and handling errors, respectively.
Code Block:
from src.utils import Utilities
from custom_logger import logger
import os
from flask import jsonify
from pptx import Presentation
import json
from flask import (
Flask,
jsonify,
render_template,
request
)
from src.prompt_templates import (
SLIDE_PLANNING_GUIDELINES,
SLIDE_CONTENT_GUIDELINES
)
import traceback
Summary:
This block imports necessary modules for utilities, logging, JSON handling, Flask web framework, and PowerPoint slide generation. The imports provide all the required functionalities to handle user interactions, generate slides, and manage sessions.
2. Flask App Initialization
Explanation:
Here, the Flask application is initialized, and the Utilities class is instantiated. This setup prepares the app to handle incoming requests and use the utility functions for session and message management.
Code Block:
app = Flask(__name__)
utils = Utilities()
Summary:
This block initializes the Flask application and creates an instance of the Utilities class, which will be used throughout the application for various helper functions, such as handling sessions and invoking language models.
3. Landing Page Route
Explanation:
The landing page route (/) renders the index.html template and passes the previous session metadata to it. This helps the user view the history of previous sessions when they access the landing page.
Code Block:
@app.route("/")
def landing_page():
previous_session_meta_data = utils.session_utils.get_session_meta_data()
return render_template("index.html", previous_session_meta_data = previous_session_meta_data)
Summary:
This route serves the landing page and provides metadata about previous sessions for the user to review. The get_session_meta_data() function from session_utils is used to fetch relevant session information.
4. Get Session Data Route
Explanation:
This route (/get_session_data) accepts a POST request with a session ID and returns the session's data. It retrieves this data using the get_session_data() function from session_utils.
Code Block:
@app.route('/get_session_data', methods=['POST'])
def get_session_data():
data = request.get_json()
session_id = data.get('sessionId')
session_data = utils.session_utils.get_session_data(session_id)
return jsonify({'session_data': session_data})
Summary:
This route fetches session data based on the provided session_id and returns it in JSON format, which is used for further processing or display.
5. Generate Slides Route
Explanation:
The /generate_slides route handles generating slides based on user input. It calls various utility functions to manage conversations, invoke the LLM (Language Model), and process slide generation. The route retrieves previous conversation messages, appends the new input, and then sends the updated conversation to an LLM to generate planning and slide content.
Code Block:
@app.route('/generate_slides', methods=['POST'])
def generate_slides():
try:
data = request.get_json()
user_input = data.get('user_input')
session_id = data.get('session_id')
# Get previous conversation messages for slide planning
messages = utils.get_previous_messages(session_id, SLIDE_PLANNING_GUIDELINES, "slides_planning")
messages.append({
"role": "user",
"content": user_input
})
logger.info(messages)
slide_planning = utils.invoke_llm(messages)
logger.info(slide_planning)
slides_content = []
for slide_details in json.loads(slide_planning)['slide_details']:
messages = utils.get_previous_messages(session_id, SLIDE_CONTENT_GUIDELINES, "slides_content")
messages.append({
"role": "user",
"content": slide_details['purpose']
})
single_slide_content = utils.invoke_llm(messages)
messages.append({
"role": "assistant",
"content": single_slide_content
})
slides_content.append(json.loads(single_slide_content))
return jsonify({'slides_content': slides_content, 'slide_planning': slide_planning}), 200
except Exception as e:
print("Error occurred:", e)
traceback.print_exc()
return jsonify({"error": "An error occurred while generating slides."}), 500
Breakdown of the Code Block:
Line 3-5: Retrieves user input and session ID from the incoming POST request.
Line 8-10: Fetches previous conversation messages related to slide planning by calling get_previous_messages()with the session ID, guidelines, and type "slides_planning".
Line 11: Appends the user's current input to the conversation history.
Line 13: Logs the updated conversation for debugging purposes.
Line 16: Sends the conversation history to the language model for slide planning, storing the result in slide_planning.
Line 19: Iterates through the slide details and fetches content for each slide.
Line 21-23: Retrieves conversation history for slide content generation, appends the slide's purpose, and sends it to the language model.
Line 27-29: Appends the assistant's response (content for the slide) to the conversation history.
Line 31: Adds the generated slide content to the slides_content list.
Line 33: Returns the generated slides content and planning as a JSON response.
Summary:
This route handles the core functionality of generating slides based on user input. It manages conversation history, interacts with an LLM, and produces both the slide planning and content, returning it as JSON.
6. Delete Session Route
Explanation:
This route (/delete_session) handles the deletion of a specific session based on the session ID provided. If no session ID is provided, it returns an error.
Code Block:
@app.route('/delete_session', methods=['POST'])
def delete_session():
data = request.get_json()
session_id = data.get('session_id')
if not session_id:
return jsonify({"error": "Session ID is required."}), 400
response, status_code = utils.session_utils.delete_session(session_id)
return jsonify(response), status_code
Summary:
This route allows for the deletion of a session based on a given session ID, ensuring the session ID is provided and using the delete_session() method from session_utils.
7. Delete All Sessions Route
Explanation:
The /delete_all_sessions route deletes all sessions. It calls the delete_all_sessions() function from session_utils.
Code Block:
@app.route('/delete_all_sessions', methods=['POST'])
def delete_all_sessions():
response, status_code = utils.session_utils.delete_all_sessions()
return jsonify(response), status_code
Summary:
This route deletes all sessions by calling the corresponding utility function, returning the result as JSON.
8. Get Thumbnails Route
Explanation:
This route (/get_thumbnails) lists all files in the static/thumbnails directory. This could be useful for displaying a list of available slide thumbnails.
Code Block:
@app.route('/get_thumbnails', methods=['POST'])
def get_thumbnails():
files = os.listdir('static/thumbnails')
return jsonify({"files": files}), 200
Summary:
This route returns a list of filenames located in the static/thumbnails directory, useful for providing thumbnails of generated slides.
9. Download Slide Route
Explanation:
This route (/download_slide) handles downloading slides. It takes a thumbnail_id and slide_data from the request and processes them.
Code Block:
@app.route('/download_slide', methods=['POST'])
def download_slide():
try:
data = request.get_json()
thumbnail_id = data.get('thumbnail_id').replace(".png", "")
slide_data = data.get('slideData')
if not thumbnail_id or not slide_data:
return jsonify({"error": "Invalid input. 'thumbnail_id' and 'slideData' are required."}), 400
return update_single_slide_ppt(thumbnail_id, transform_slide_data(slide_data))
except Exception as e:
print("Error occurred:", e)
traceback.print_exc()
return jsonify({"error": "An error occurred while generating slides."}), 500
Summary:
This route handles the downloading of a slide based on the provided thumbnail_id and slide_data. It uses update_single_slide_ppt() to update the PowerPoint slide.
10. Update Session Route
Explanation:
The /update_session route updates a session with new slide planning and content. It calls the add_slides() function from session_utils.
Code Block:
@app.route('/update_session', methods=['POST'])
def update_session():
data = request.get_json()
session_id = data.get('session_id')
prompt = data.get('prompt')
slide_planning = data.get('slide_planning')
slides_content = json.dumps(data.get('slides_content'))
utils.session_utils.add_slides(session_id, prompt, slide_planning, slides_content)
return jsonify({"success" : True})
Summary:
This route updates the session with new slide-related data (planning and content), calling the utility function add_slides() to persist the changes.
11. Running the App
Explanation:
The app is run on 0.0.0.0 and port 8000, making it accessible to all users on the network.
Code Block:
if __name__ == "__main__":
app.run(host="0.0.0.0", port=8000)
Summary:
This block ensures the Flask app runs and listens for incoming requests.
Conclusion
In this blog post, we explored the Gen AI PPT Maker, a project that leverages AI to create PowerPoint presentations based on user input. By utilizing OpenAI's GPT models, Flask, and PowerPoint templates, the project enables users to generate professional presentations with ease.
If you enjoyed this project, feel free to explore more of my work on my website and YouTube channel. Don't forget to check out other tutorials and projects that will help enhance your skills in data science and AI!
Follow me on LinkedIn: Sai Ram Penjarla
Follow me on Instagram: @sairam.ipynb
Happy Coding!